Hi, there! I would like to cut a piece of the world to work with, how can i do that? Like the map of my game is just one city, not the whole world. Thanks!
You can implement a tile excluder:
CesiumGS:main
← CesiumGS:tile-excluder
opened 06:03AM - 16 Mar 23 UTC
Depends on CesiumGS/cesium-native#608
Added `CesiumTileExcluder`, which is a … C# version of cesium-native's `ITileExcluder`.
The idea is that we can add a C# class like this to our project:
```csharp
using CesiumForUnity;
using UnityEngine;
[RequireComponent(typeof(BoxCollider))]
public class CesiumBoxExcluder : CesiumTileExcluder
{
private BoxCollider _boxCollider;
private Bounds _bounds;
public bool invert = false;
protected override void OnEnable()
{
this._boxCollider = this.gameObject.GetComponent<BoxCollider>();
this._bounds = new Bounds(this._boxCollider.center, this._boxCollider.size);
base.OnEnable();
}
protected void Update()
{
this._bounds.center = this._boxCollider.center;
this._bounds.size = this._boxCollider.size;
}
public bool CompletelyContains(Bounds bounds)
{
return Vector3.Min(this._bounds.max, bounds.max) == bounds.max &&
Vector3.Max(this._bounds.min, bounds.min) == bounds.min;
}
public override bool ShouldExclude(Cesium3DTile tile)
{
if (!this.enabled)
{
return false;
}
if (this.invert)
{
return this.CompletelyContains(tile.bounds);
}
return !this._bounds.Intersects(tile.bounds);
}
}
```
And then add an instance of this class as a component on the `Cesium3DTileset` or any of its parents, up to and including the `CesiumGeoreference`.
With that in place, cesium-native will call the `ShouldExclude` method for each tile that it is considering loading or rendering. This will happen a _lot_, so this method needs to be fast. Return true to skip loading and rendering that tile. Return false to load it and render it as normal.
In the implementation above, a user-defined BoxCollider is also attached to the same GameObject as the CesiumBoxExcluder. Any tiles that intersect this BoxCollider are loaded and rendered, others are not. So tiles that are entirely outside of the box are ignored. The given tile's `bounds` property is an axis-aligned bounding box in the same coordinate system as the `CesiumBoxExcluder`, so it's easy to do tests like this.
In this screenshot, the BoxCollider is the green box in the middle of Melbourne. All of the visible tiles are at least partially inside the box:
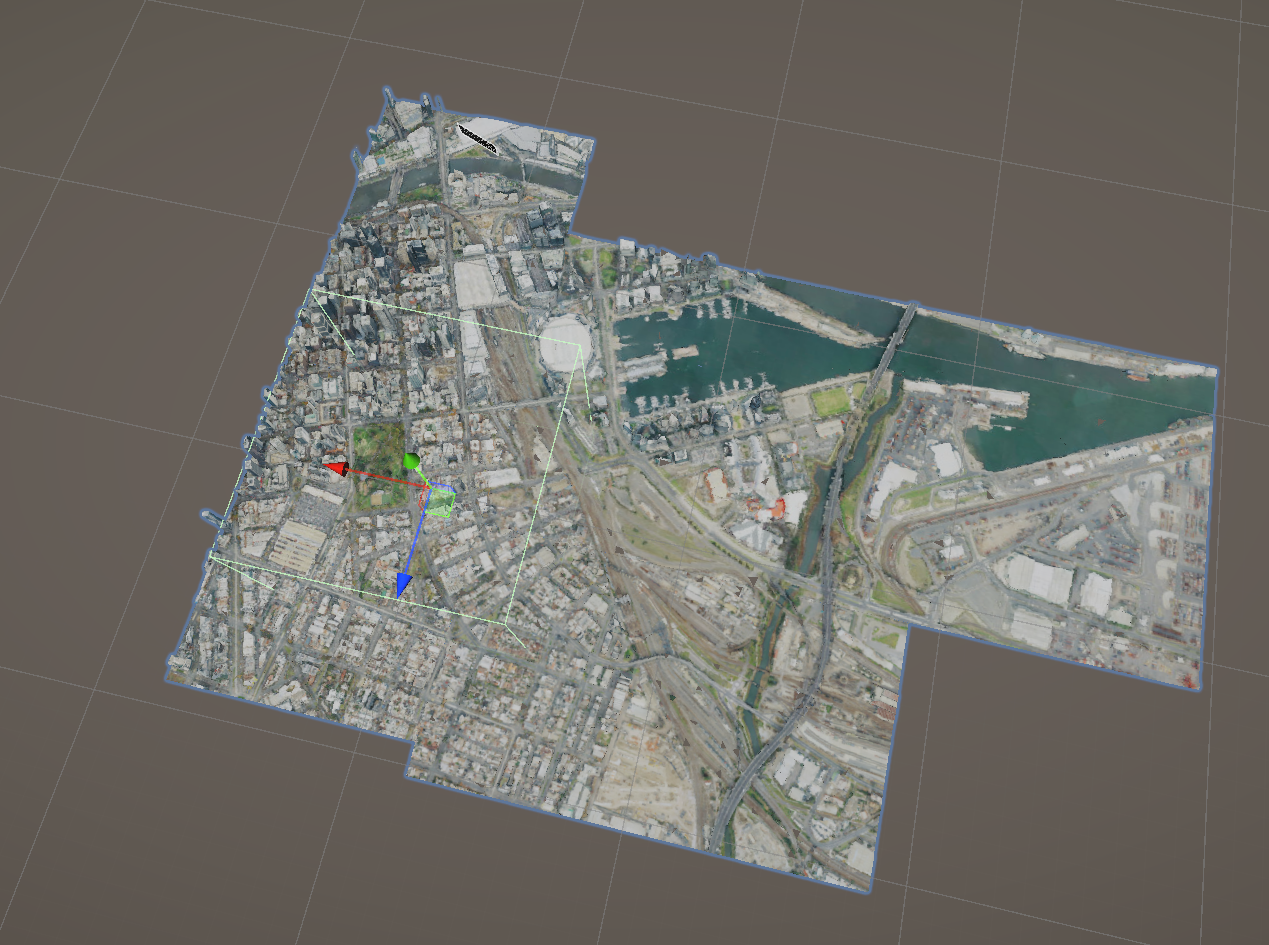
If we disable the CesiumBoxExcluder, all the tiles load as normal:
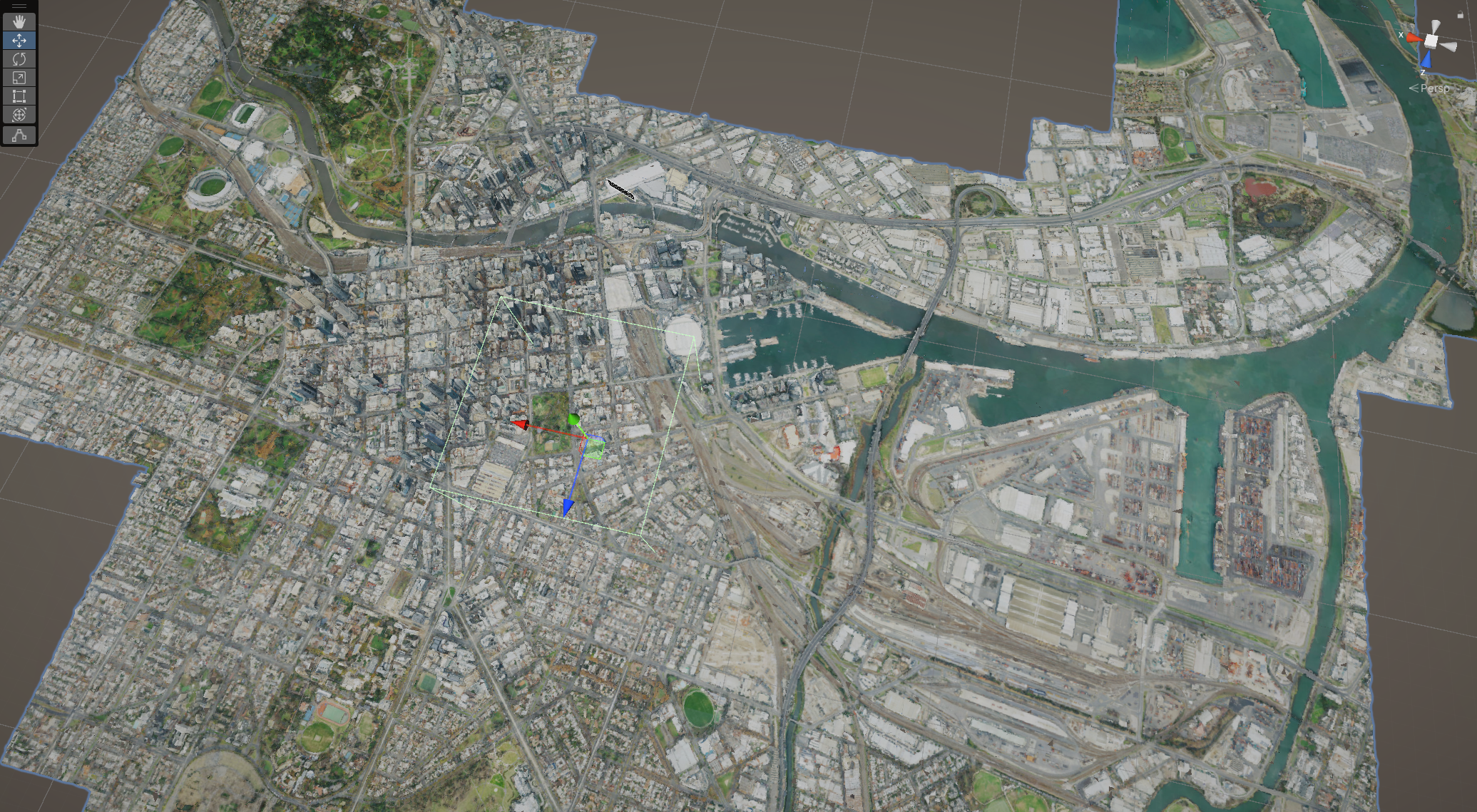
Also in this PR: Extended Reinterop to allow constructors of blittable value types to be called from C++. On the C++ side, these are exposed as `Constrct` methods rather than C++ constructors so that brace initialization can continue to be used to initialize fields without calling into C#.
1 Like